How to Redirect to Another Page in JavaScript on Button Click?
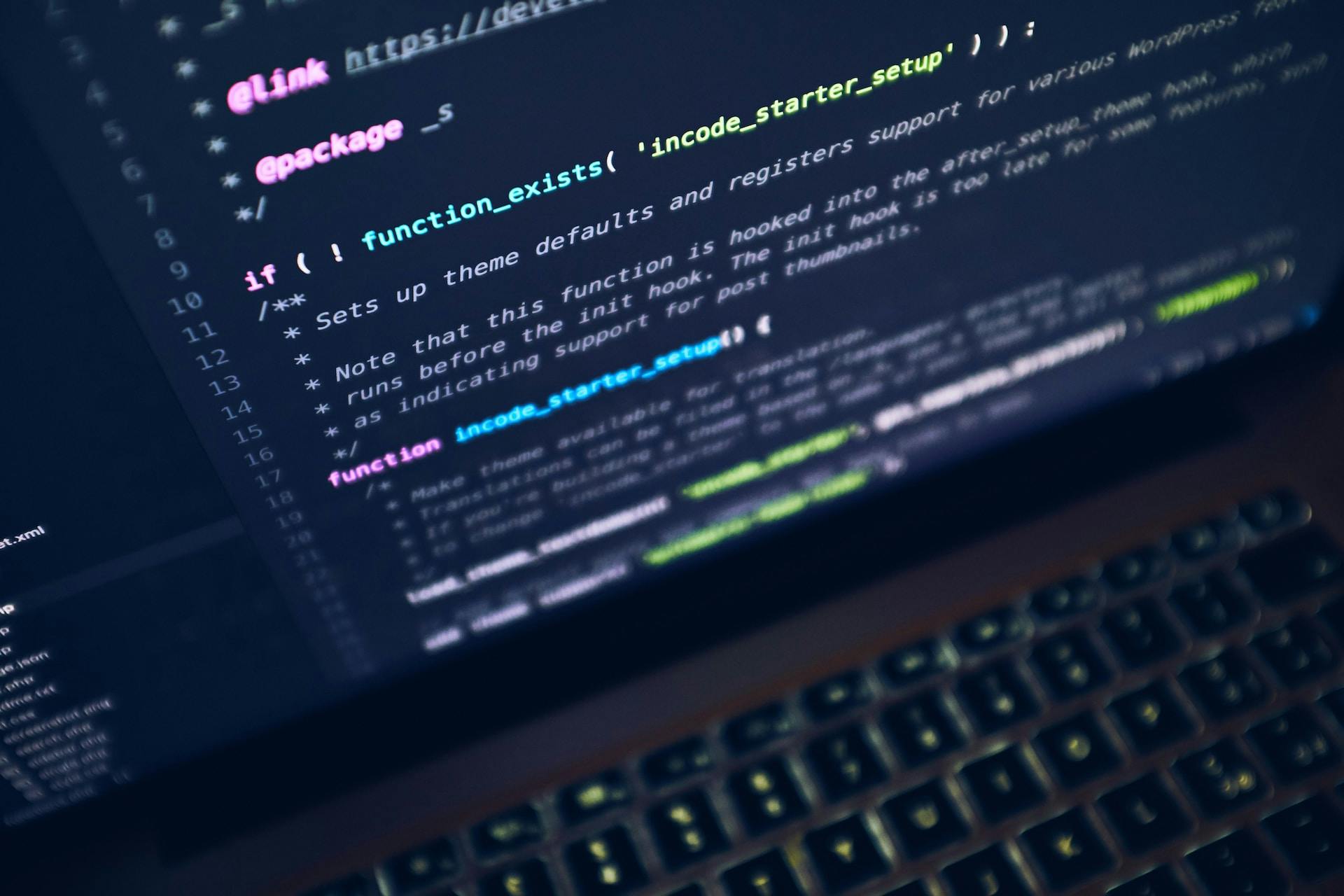
Table Of Content
- What is Redirecting?
- What is this problem?
- Let's recreate an example
- Code example
- Alternative Solutions
- Solution 1: Using a Function
- Solution 2: jQuery Approach
- Solution 3: Inline onclick Attribute
- Solution 4: Using a Link Element (Without Target)
- Solution 5: Using a Link Element (With Target=_blank)
- Solution 6: Redirect using HTML Form Tag (GET)
- Solution 7: Redirect using HTML Form Tag (POST)
- Solution 8: Using `location.replace`
- FAQs
- Why isn't my button click redirecting to the specified page?
- When should I use a link element over a button for redirection?
- Can I open the redirection in a new tab or window?
- Are there any considerations for styling links as buttons?
- How can I navigate within the same website without a full page reload?
- Conclusion
- References
In the dynamic landscape of web development, one common requirement involves seamlessly redirecting users to different pages based on specific interactions, such as button clicks. To embark on this journey, it's crucial to understand what redirection means and then explore simple yet effective solutions using JavaScript
, HTML
, and jQuery
.
What is Redirecting?
Redirecting, in web development terms, refers to the process of automatically sending a user from one web page to another. This mechanism plays a pivotal role in crafting intuitive and user-friendly navigation experiences within websites and web applications.
What is this problem?
The challenge lies in creating a button
that, upon clicking, triggers the seamless redirection to another page. This task is pretty simple.
Let's recreate an example
Let's begin with a basic HTML
file containing a button
element and an attempt to use JavaScript
to handle the redirection. This is a straightforward approach.
Code example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Redirect Example</title>
</head>
<body>
<h1>Simple redirect Example</h1>
<p>Click me to redirect to another Page</p>
<button id="redirectButton">Click to Redirect</button>
<script>
document.getElementById('redirectButton').addEventListener('click', function() {
window.location.href = 'https://www.example.com';
});
</script>
</body>
</html>
Lets see what else we can do for this simple task.
Alternative Solutions
Solution 1: Using a Function
<script>
function redirectToExample() {
window.location.href = 'https://www.example.com';
}
document.getElementById('redirectButton').addEventListener('click', redirectToExample);
</script>
Use this approach when you want a clear and organized separation of concerns. This method is advantageous for maintaining readability and reusability in your code.
Solution 2: jQuery Approach
Ensure the jQuery
library is included:
<script src="https://code.jquery.com/jquery-3.6.4.min.js"></script>
Then use the following code:
<script>
$(document).ready(function(){
$('#redirectButton').click(function(){
window.location.href = 'https://www.example.com';
});
});
</script>
Opt for jQuery
when you are already utilizing the library in your project. This solution streamlines event handling and is beneficial if you're comfortable with jQuery
syntax.
Solution 3: Inline onclick Attribute
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Redirect Example</title>
</head>
<body>
<h1>Simple redirect Example</h1>
<p>Click me to redirect to another Page</p>
<button id="redirectButton" onclick="window.location.href='https://www.example.com';">Click to Redirect</button>
</body>
</html>
Choose this approach for simplicity in cases where the redirection logic is concise. However, be cautious with this method for larger projects to maintain maintainability.
Solution 4: Using a Link Element (Without Target)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Redirect Example</title>
</head>
<body>
<h1>Simple redirect Example</h1>
<p>Click me to redirect to another Page</p>
<a href="https://www.example.com" id="redirectButton" style="display: inline-block; padding: 10px 20px; background-color: #4CAF50; color: white; text-align: center; text-decoration: none; display: inline-block; font-size: 16px; margin: 4px 2px; cursor: pointer; border-radius: 4px;">Click to Redirect</a>
</body>
</html>
Use a link element when your button serves a navigation purpose and does not require additional JavaScript
. This is suitable for basic scenarios with straightforward navigation needs.
The added style attribute provides a button-like appearance.
When styling links to look like buttons, use the
style
attribute or, preferably, define a class in yourCSS
for consistent styling.
Avoid using inline styles extensively for larger projects; prefer external
CSS
files for better maintainability.
Solution 5: Using a Link Element (With Target=_blank)
Consider user experience when deciding between opening links in the same tab or a new tab.
<a href="https://www.example.com" target="_blank" id="redirectButton">Click to Redirect (New Tab)</a>
Opt for this approach when you want the redirection to open in a new tab or window. This is helpful when you want to retain the original page for reference.
Solution 6: Redirect using HTML Form Tag (GET)
<form action="/search" method="get">
<label for="searchQuery">Search:</label>
<input type="text" id="searchQuery" name="q">
<button type="submit">Search</button>
</form>
Choose the form tag with the GET method when you want to initiate redirection through form submission. This is suitable for scenarios where you need to include parameters in the URL.
Solution 7: Redirect using HTML Form Tag (POST)
<form action="/login" method="post">
<label for="username">Username:</label>
<input type="text" id="username" name="username">
<label for="password">Password:</label>
<input type="password" id="password" name="password">
<button type="submit">Login</button>
</form>
Select the form tag with the POST method when you need to perform a more secure or data-sensitive redirection. Use this when you want to send data to the server without exposing it in the URL.
Solution 8: Using location.replace
<script>
document.getElementById('redirectButton').addEventListener('click', function() {
window.location.replace('https://www.example.com');
});
</script>
Opt for location.replace
when you want to replace the current page in the browser's history. This is beneficial for scenarios where you want to avoid the original page being accessible through the browser's back button.
FAQs
Why isn't my button click redirecting to the specified page?
This issue could be caused by various factors, such as script execution timing, conflicts with other scripts, or browser-specific behaviors. Consider using a function or the jQuery approach to ensure proper event handling.
When should I use a link element over a button for redirection?
Use a link element when the button primarily serves a navigation purpose and does not require additional JavaScript. This is suitable for basic scenarios with straightforward navigation needs.
Can I open the redirection in a new tab or window?
Yes, you can achieve this by using the target="_blank"
attribute in a link element or by using JavaScript with window.open()
.
Are there any considerations for styling links as buttons?
When styling links to look like buttons, ensure consistent styling using CSS, either through the style
attribute or a defined class. Consider accessibility and provide alternative visual cues for users with disabilities.
How can I navigate within the same website without a full page reload?
Use JavaScript to handle the click event of a link, prevent the default behavior using event.preventDefault()
, and update the page content dynamically without a full reload.
Conclusion
Effectively redirecting users on button click is a common and crucial task in web development. By understanding the concept of redirection and exploring diverse solutions, including the use of functions, jQuery
, inline onclick attributes, link elements (with and without target), HTML
form tags (GET and POST), and the location.replace
method, you can ensure a smoother and more reliable redirection experience for users across various scenarios. Experiment with these solutions based on your specific project requirements to enhance user navigation on your website or web application.